Some developers come to TypeScript after programming in JavaScript for years. As a result, in a hurry to get the job done,
some of these developers do not take full advantage of the more advanced object-oriented features of TypeScript.
This is understandable in 2024. The claim of putting the code into production can be rigid and unreliable.
Taking the time to fully learn the principles of object-oriented programming in the context of TypeScript becomes a luxury. There is a lot to know.
However, some essential principles of object-oriented programming can not only be learned quickly but will also save time when creating and maintaining applications.
One set of principles is to promote the virtuous use, security, and versatility of code using interfaces and abstract classes.
In object-oriented programming, both abstract classes and interfaces serve as fundamental constructs for defining context.
They create an account for other classes, ensuring a consistent work statement of behaviors and behaviors. However, they each come with different features and use cases.
So always we will know the complete information in the article, stay tuned till the end.
Difference Between Interface and Abstract Class
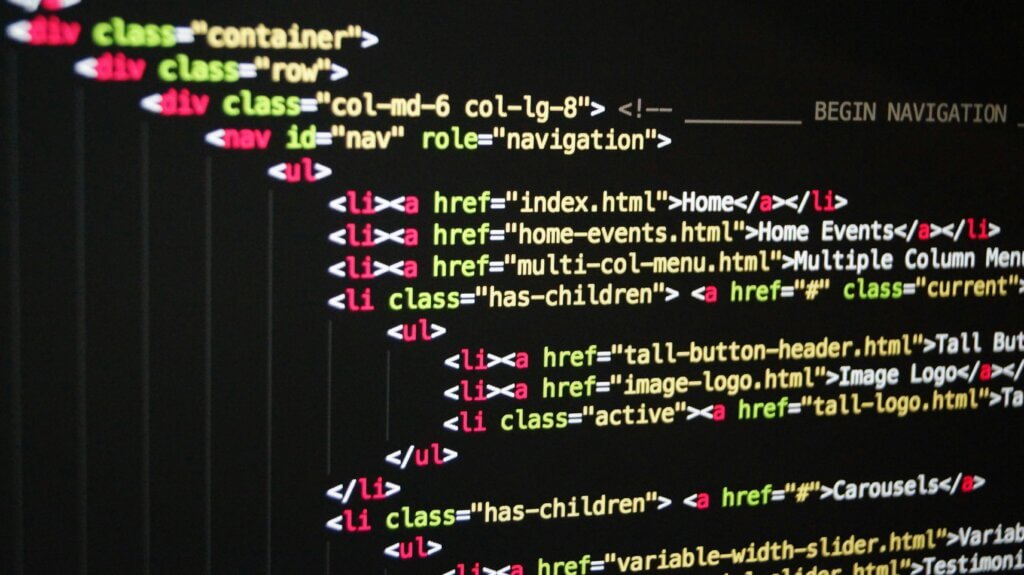
As we know abstraction means to hide the internal workings of the feature and show only the functionality to users i.e.
show only the essential features and hide how all those features are implemented behind the scenes whereas interface is another way to achieve abstraction in Java abstract class and interface both are used for abstraction so the interface and abstract class are the necessary terms
Abstract Class in Java
1. Definition: An abstract class is a class that cannot be instantiated directly. It acts as a blueprint for other classes
2. Method Implementation: An abstract class can contain both methods without a function statement and abstract methods
3. Variables: abstract classes can have members that include final non-final level and non-local variables
4. Constructors: abstract classes can have constructors which are used to initialize variables in the abstract class when passed by the parent class
Read This Article To Know More:- What Is The Difference Between Null Undefined And Undeclared In JavaScript?
Exmaple1:
JAVA
abstract class Shape {
String name;
Shape(String name) {
this.name = name;
}
public void moveTo(int x, int y) {
System.out.println(name + " moved to position x = " + x + ", y = " + y);
}
public abstract double calculateArea();
public abstract void render();
}
class Rectangle extends Shape {
int length, width;
Rectangle(int length, int width, String name) {
super(name);
this.length = length;
this.width = width;
}
@Override
public void render() {
System.out.println("Drawing a rectangle.");
}
@Override
public double calculateArea() {
return length * width;
}
}
class Circle extends Shape {
final double PI = 3.14159;
int radius;
Circle(int radius, String name) {
super(name);
this.radius = radius;
}
@Override
public void render() {
System.out.println("Drawing a circle.");
}
@Override
public double calculateArea() {
return PI * radius * radius;
}
}
public class Main {
public static void main(String[] args) {
Shape rectangle = new Rectangle(2, 3, "Rectangle");
System.out.println("Rectangle area: " + rectangle.calculateArea());
rectangle.moveTo(1, 2);
System.out.println();
Shape circle = new Circle(2, "Circle");
System.out.println("Circle area: " + circle.calculateArea());
circle.moveTo(2, 4);
}
}
Output
Area of rectangle: 6.0
Rectangle has been moved to x = 1 and y = 2
Area of circle: 12.56
Circle has been moved to x = 2 and y = 4
Example 2:
// Abstract class Sunstar
abstract class Sunstar {
// Abstract method printInfo
abstract void printInfo();
}
// Employee class that extends the abstract class Sunstar
class Employee extends Sunstar {
private String name; // Employee name
private int age; // Employee age
private float salary; // Employee salary
// Constructor to initialize Employee details
public Employee(String name, int age, float salary) {
this.name = name;
this.age = age;
this.salary = salary;
}
// Implementation of the abstract method printInfo
@Override
void printInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("Salary: " + salary);
}
}
// Base class to test the implementation
public class Base {
public static void main(String[] args) {
// Create an Employee object with specific details
Sunstar s = new Employee("Avinash", 21, 222.2F);
s.printInfo(); // Call the printInfo method
}
}
Output
Avinash
21
222.2
Interface in Java
1. Definition: An interface in Java is a reference type. It is similar to a class and is a collection of abstract methods and constants.
2. Method Implementation: All methods in an interface are constants by default and must be implemented by any class that implements the interface.
From Java 8 onwards, interfaces can have default and constant methods with concrete functions. From Java 9 onwards, interfaces can also have private methods.
3. Variables: Methods declared in an interface are public and final constants by default.
Example 3:
JAVA
interface Drawable {
void draw();
}
interface Movable {
void moveTo(int x, int y);
}
class Circle implements Drawable, Movable {
private static final double PI = 3.14;
private int radius;
private int x, y;
Circle(int radius, int initialX, int initialY) {
this.radius = radius;
this.x = initialX;
this.y = initialY;
}
@Override
public void draw() {
System.out.println("Drawing a circle with radius " + radius + " at position (" + x + ", " + y + ")");
}
@Override
public void moveTo(int x, int y) {
this.x = x;
this.y = y;
System.out.println("Circle moved to position (" + x + ", " + y + ")");
}
public double getCircumference() {
return 2 * PI * radius;
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle(2, 0, 0);
circle.draw();
circle.moveTo(2, 4);
circle.draw(); // To see the updated position after moving
System.out.println("Circumference of the circle: " + circle.getCircumference());
}
}
Output
Circle has been drawn
Circle has been moved to x = 2 and y = 4
Abstract class vs Interface
Features of an Abstract Class in Java
An abstract class in Java is a special type of class that cannot be instantiated directly. It acts as a reference for other classes thereby defining a common interface or behaviour that different classes can share.
However each derived class has its own shared features. This will make this premise more clear.
Cannot be instantiated: abstract classes cannot be instantiated directly which means you cannot create objects of the abstract class.
This means that the abstract class only acts as a template that other classes can extend but it cannot be an instance of itself.
Combination of partial implementation and subclass contract: abstract methods in abstract classes can be methods that have no implementation statements that must be overwritten by subclasses.
Additionally, Guava classes can also have concrete methods with implementation statements. This combination allows abstract classes to have partial implementations and a contract for derived classes to follow.
This allows derived classes to ensure that they implement the required functionality.
Can have both abstract and non-abstract methods: abstract classes can have both abstract and non-guava methods.
Non-guava methods are purely function requests and can be called directly. Thus abstract classes not only define the behavior needed by subclasses but also provide abstract implementation of some functionality.
Can have constructors: abstract classes can have constructors that are called when their subclasses are instantiated.
This allows for the initial setup or initialization of the required class fields during the creation of subclass objects even if no instance of the abstract class can be created.
Can have member variables: abstract classes can have member variables that are related to the class instance. These member variables can be final, non-final, static, or non-static. They can be inherited or modified by subclasses.
Use as a base class: abstract classes can be used as base classes for other classes. This means that other classes can inherit them.
This encourages the virtuous use of code. and provides a uniform interface or behavior for related classes, creating an organized and coherent system
Read This Article To Know More:- What Is The Key Difference Between An Abstract Class And An Interface In TypeScript?
Features of an Interface in Java
An interface in Java is a reference type that is similar to a class but can contain only constants, method signatures, default methods, state methods, and nested types.
An interface cannot have instance fields and its methods are static by default except for default and static methods introduced in Java 8 and private methods introduced in Java 9.
This feature of interfaces makes it particularly useful when there is a need to define a contract or behavior between different classes in which it is bound to provide function statements of all the methods defined by the interface.
Definition and Functionality of Interface: An interface defines a set of static fields and functions.
Any class implementing an interface is responsible for its function statements. It acts as a contract that ensures that the class has the required methods defined by the interface.
Also, constants such as public static final char can be declared in interfaces making some of the methods invariant.
Common Protocol and Communication: An interface is to establish a common protocol between software components allowing them to communicate with each other.
It ensures consistency and interoperability across different parts of the software system allowing seamless communication and collaboration between different components
Support Polymorphism: An interface supports polymorphism which identifies objects of different classes as a common interface type.
As long as the objects implement the respect interface they can be used interchangeably with flexibility and extensibility which makes the use and maintenance of code easier
Ability to separate concerns: Interfaces facilitate separation of concerns by defining the signature of methods and making them independent of their function functions.
It allows different parts of a software system to be developed independently as long as they follow the specified rules of the interface.
It helps in keeping the function and details separate from the interface which makes the structure of the code clearer
Improves code reusability: Interfaces improve code reusability as it allows different classes to implement the same interface.
This means that the common functionality defined by the interface can be used across multiple classes which makes the code more efficient The work will be done by default and maintenance will be easy
Compliance with design patterns and best practices: Interfaces help to implement certain design patterns which can be done through adapter patterns or strategy patterns or the necessary interfaces and folds to the interface thereby ensuring the design conforms to best practices and compliance
Facilitates testing: Interfaces also simplify the testing process as they allow the use of mock objects that invoke the interface.
This allows software components to be tested independently of each other ensuring that each component functions correctly in isolation before being integrated into a larger system
New features of interfaces in Java 8 and 9: Since Java 8, interfaces can include default methods that come with the function statement.
This feature provides a way to add new methods to the interface thereby extending the interface without breaking the existing framework.
Apart from this, interfaces can also have default methods that are associated with the entire interface rather than a particular instance.
Since Java 9, interfaces can also include private methods which are used to test interfaces. The defaults help to further encapsulate shared code between videos, thereby avoiding code waste and making the internal structure of the interface more efficient
When to use what?
Consider using Ambrosia classes if any of the following statements apply to your situation:
Parallelism in Java Applications: If you have a few related classes in a Java application that need to share a few lines of code,
you can put those common code lines within an Ambrosia class. These related classes can then extend this Ambrosia class, making the code easier to use and maintain.
Fields and Methods: Within an Ambrosia class, you can define final and non-final fields so that the state of the related object can be accessed and modified through a single method. This allows you to centralize shared business between classes.
Common methods and access modifiers: Using a Guava class is advisable when you expect that the classes that extend Ambrosia will have many common methods or fields, or when you need access modifiers other than public, protected, and private.
Consider using a Rails interface if any of the following statements may apply to your situation:
Full Encapsulation: The pipeline provides a complete encapsulation that includes all the requirements to be implemented by the class implementing this pipeline. It allows specifying a related data behavior explicitly.
Multiple Inheritance: More than one class can implement a portfolio which is called multiple inheritance. Thus the behaviours of different portfolios can be accommodated in a single class.
Behavior Unified: The pipeline is effectively used when you want to ensure your behavior is the same without worrying about the function congruence of a data type.
Read This Article To Know More:- What Is The Key Difference Between An Abstract Class And An Interface In TypeScript?
Conclusion
Abstract classes in Java serve as blueprints that cannot be instantiated directly. These classes can contain both concrete and concrete methods and allow partial implementations.
Guava classes can have any number of member classes that can have any number of access parameters.
In contrast, interfaces are contracts that specify a set of Guava methods that a class must implement.
However, since Java 8, interfaces can also contain default and return methods. They implement polymorphism and implement a common protocol for different software components.
Unlike abstract classes, all interface methods are inherently public and their parameters are always public and final.
FAQs
What is the main difference between type and interface in TypeScript?
In TypeScript, the main difference is that type
can define a union or intersection of types and supports more complex type compositions, while interface
is primarily used for defining the shape of objects and supports declaration merging.
What is the difference between an abstract data type and an interface?
An abstract data type (ADT) defines a data structure and its operations abstractly, focusing on what operations are performed rather than how they’re implemented. An interface, on the other hand, specifies a contract for what methods a class must implement but doesn’t dictate how those methods are implemented.